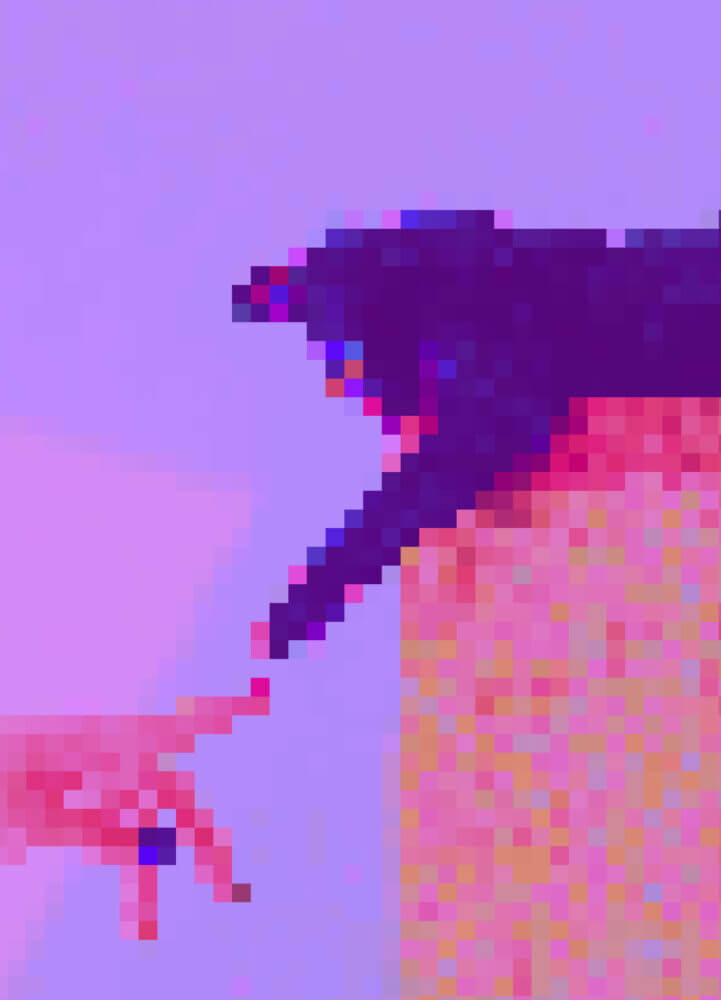
A quick post on how to improve your Gutenberg WP theme by fixing a pesky issue with the core blocks stylesheet: it’s…*ahum* opinionated and in a lot of places difficult to override.
And adding more and more CSS to your site for every little tweak you need is just bad practice (inline CSS stylesheets, I’m looking at you). So what is a humble dev to do?
Luckily I learned – from a comment on Github burried in a thread on the problem of the default Gutenberg block breakpoints (that’s a mouthful) – that you can remove styles of core blocks on the individual block level.
Being able to replace only styles of specific blocks is essential because that means it’s not an all or nothing option: you don’t need to redo – and maintain – your own copy of the full blocks stylesheet.
How it works
- output the default CSS block styles as separate styles per block with the should_load_separate_core_block_assets filter.
As per the docs: this function checks whether separate styles should be loaded for core blocks on-render. - you can then deregister the block styles you don’t want per core block with the function wp_deregister_style which removes a registered stylesheet.
- you can now happily provide your own styles for the core blocks of your choice.
That’s it!
Use case: custom breakpoints for the post template – query loop block
Here’s an example where I used this in one of my latest projects: I reset the query loop breakpoints.
I don’t like the 600px breakpoint the default block styles use: it’s far too narrow and makes your site look like shit on tablet. I’m not kidding, take a look at this:
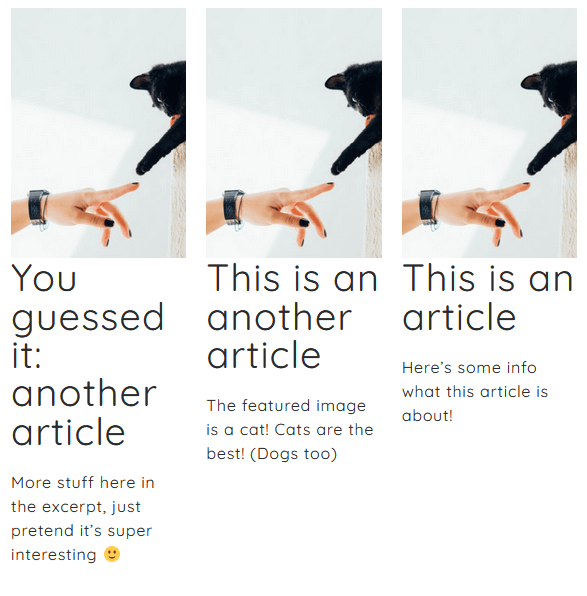
Yeah, that needed to change.
1. Adding the functions
First step: add the code below to the theme’s functions.php. This will load the core block styles as separate assets and de-register the ones you want to remove.
In this case the block name is post-template (this is the query loop block in the editor, yes the naming is somewhat confusing).
// Gutenberg - load block styles as separate assets
add_filter( 'should_load_separate_core_block_assets', '__return_true' );
// Gutenberg - deregister individual block styles
function use_custom_styles() {
// add the name(s) of the specific block styles you want to deregister
// keep in mind that some blocks like the columns block consist out of multiple blocks
$blocks = array(
'post-template'
);
foreach ( $blocks as $block ) {
wp_deregister_style( 'wp-block-' . $block );
//note that you could also register a specific stylesheet per block like so:
// wp_register_style( 'wp-block-' . $block, 'path/to/custom/block.css' );
}
}
add_action( 'wp_enqueue_scripts', 'use_custom_styles' );
Not sure which styles you need to override and what the block name is?
Once you load the block styles separately with should_load_separate_core_block_assets you can view them in the source code of the header (for each block that is currently on the page).
Drop the block you want to restyle in a page, inspect the source and you can get the correct name and the bits of CSS you need to re-write.
2. Styling with CSS/SCSS
Next up: load new CSS styles for the blocks in the theme’s stylesheet (or add a separate block style to be enqueued with wp_register_style, that’s up to you).
Here’s what that looks like:
.wp-block-post-template, .wp-block-query-loop {
margin-top: 0;
margin-bottom: 0;
max-width: 100%;
list-style: none;
padding: 0;
}
.wp-block-post-template.wp-block-post-template, .wp-block-query-loop.wp-block-post-template {
background: none;
}
.wp-block-post-template.is-flex-container, .wp-block-query-loop.is-flex-container {
display: -ms-grid;
display: grid;
gap: 1.2em;
}
.wp-block-post-template li, .wp-block-query-loop li {
margin: 0;
width: 100% !important;
}
/* set a new breakpoint for phablet/tablet screens */
@media (min-width:783px) {
.wp-block-post-template.columns-2, .wp-block-post-template.columns-3, .wp-block-post-template.columns-4, .wp-block-post-template.columns-5, .wp-block-post-template.columns-6, .wp-block-query-loop.columns-2, .wp-block-query-loop.columns-3, .wp-block-query-loop.columns-4, .wp-block-query-loop.columns-5, .wp-block-query-loop.columns-6 {
-ms-grid-columns: (1fr)[2];
grid-template-columns: repeat(2, 1fr);
}
}
/* set a new breakpoint for desktop screens */
@media (min-width:1251px) {
.wp-block-post-template.columns-3, .wp-block-query-loop.columns-3 {
-ms-grid-columns: (1fr)[3];
grid-template-columns: repeat(3, 1fr);
}
.wp-block-post-template.columns-4, .wp-block-query-loop.columns-4 {
-ms-grid-columns: (1fr)[3];
grid-template-columns: repeat(3, 1fr);
}
.wp-block-post-template.columns-5, .wp-block-query-loop.columns-5 {
-ms-grid-columns: (1fr)[3];
grid-template-columns: repeat(3, 1fr);
}
.wp-block-post-template.columns-6, .wp-block-query-loop.columns-6 {
-ms-grid-columns: (1fr)[3];
grid-template-columns: repeat(3, 1fr);
}
}
Yes, it uses CSS grid now! And it always breaks down to 2 columns on smaller screens if the column count is 3 or more, before collapsing all the way to a single column.
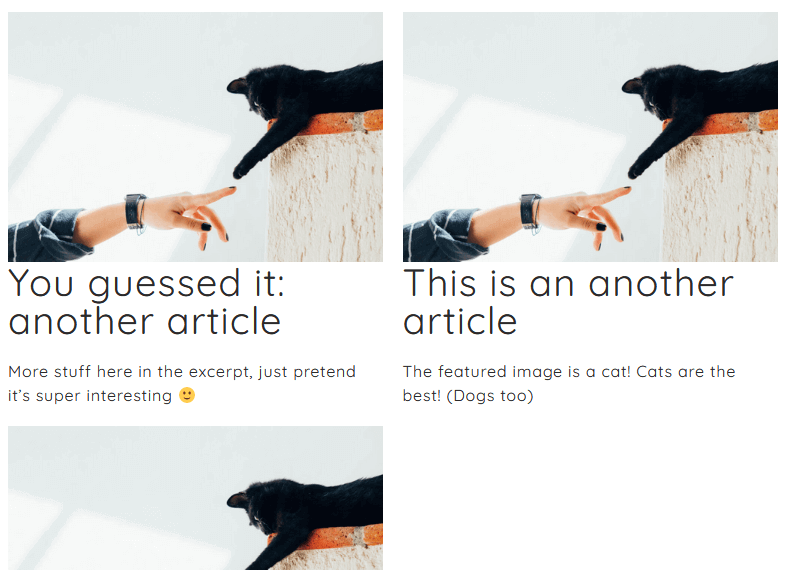
Obviously this is an opinionated design choice too, but it’s my choice, which – I figure – is the point of designing a theme to begin with.
✨ I recommend copying the core blocks stylesheet CSS and using it as a reference to make sure you don’t miss any of the now myriad of block settings.
Considerations
So are there downsides to this method you ask? Well, this doesn’t work in the editor as far as I know. 🤷♀️ I can live with that.
I’ve only tested this method for block themes, not hybrid themes (meaning: Gutenberg enabled themes without a theme.json) but I don’t think this should be a problem. Let me know if you test this and can verify it works for hybrid themes too.
Is it future proof? I can’t guarantee anything when it comes to Gutenberg development but I’m optimistic. The should_load_separate_core_block_assets function was introduced in 5.8 so it would be odd if support would drop for it immediately, especially because it serves several purposes in the WP script loader. De-registering styles is a standard WP functionality. Overall I think it’s a safe bet this will continue to work in the future.
Cute cat image by Humberto Arellano on Unsplash